TestNG Tutorial
TestNG Annotations
What Are TestNG Annotations ?
TestNG annotations are the set of codes that control the order of the execution of methods and enable the tests to run in an organized and defined manner.
It is necessary to annotate each method in testNG to run the test otherwise testNG will ignore the method which have no annotation since it won't know when to execute this method.
An annotaion in testNG starts from '@' and follows the annotaion name which is predefined.
Before going further let's discuss some important terminologies related to it :
Suite : It has one or more tests.
Test : It has one or more classes.
Class : It has one or more methods.
Types of TestNG Annotations
TestNG has a variety of annotations that are of ten kinds as follows :
@BeforeSuite : Runs just once before the execution of all the test methods.
@AfterSuite : Runs just once after the execution of all the test methods.
@BeforeTest : Runs just once before the execution of all the test methods that are within same test class.
@AfterTest : Runs just once after the execution of all the test methods that are within same test class.
@BeforeClass : Runs just once before the first method invokes of the current class.
@AfterClass : Runs once just after the execution of all test methods of the current class.
@BeforeMethod : Runs each time before a test method executes.
@AfterMethod : Runs each time after a test method executes.
@BeforeGroups : Runs just once before the execution of tests of that group.
@AfterGroups : Runs just once before the execution of tests of that group.
Hierarchy of the TestNG Annotations
@BeforeSuite
@BeforeTest
@BeforeClass
@BeforeMethod
@Test
@AfterMethod
@AfterClass
@AfterTest
@AfterSuite
To explore more let's understand the annotaions with an example :
package testNGexamples;
import org.testng.annotations.Test; import org.testng.annotations.BeforeMethod; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeClass; import org.testng.annotations.AfterClass; import org.testng.annotations.BeforeTest; import org.testng.annotations.AfterTest; import org.testng.annotations.BeforeSuite; import org.testng.annotations.AfterSuite;
public class TestNGAnnotaions {
@Test public void Test1() { System.out.println("Test 1 is executing..."); } @Test public void Test2() { System.out.println("Test 2 is executing..."); }
@BeforeMethod public void beforeMethod() { System.out.println("Before Method is executing..."); }
@AfterMethod public void afterMethod() { System.out.println("After Method is executing..."); }
@BeforeClass public void beforeClass() { System.out.println("Before Class is executing..."); }
@AfterClass public void afterClass() { System.out.println("After Class is executing..."); }
@BeforeTest public void beforeTest() { System.out.println("Before Test is executing..."); }
@AfterTest public void afterTest() { System.out.println("After Test is executing..."); }
@BeforeSuite public void beforeSuite() { System.out.println("Before Suite is executing..."); }
@AfterSuite public void afterSuite() { System.out.println("After Suite is executing..."); }
}
Output :
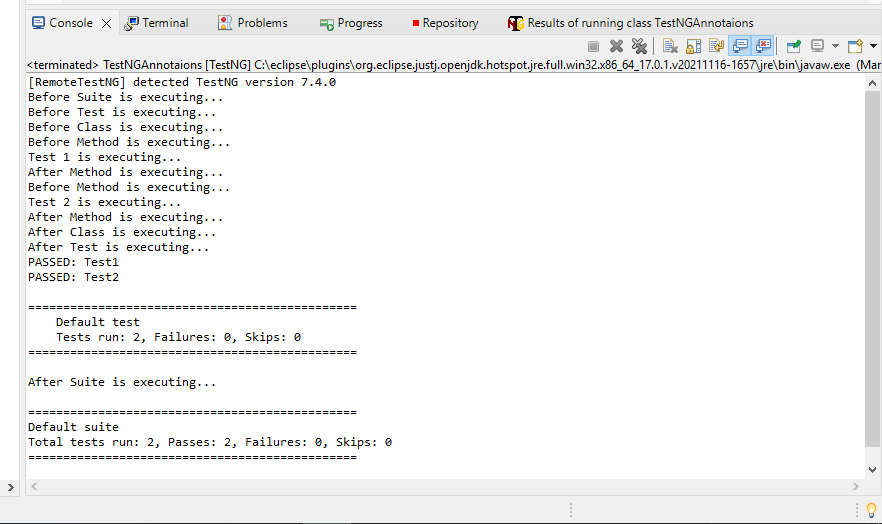